If you’re serious about your coding standards, you’re probably using the latest and greatest C++ features. For this reason, you need to set your compiler’s C++ standards to whatever new version you need. In this post, we will set the C++ standard with CMake.
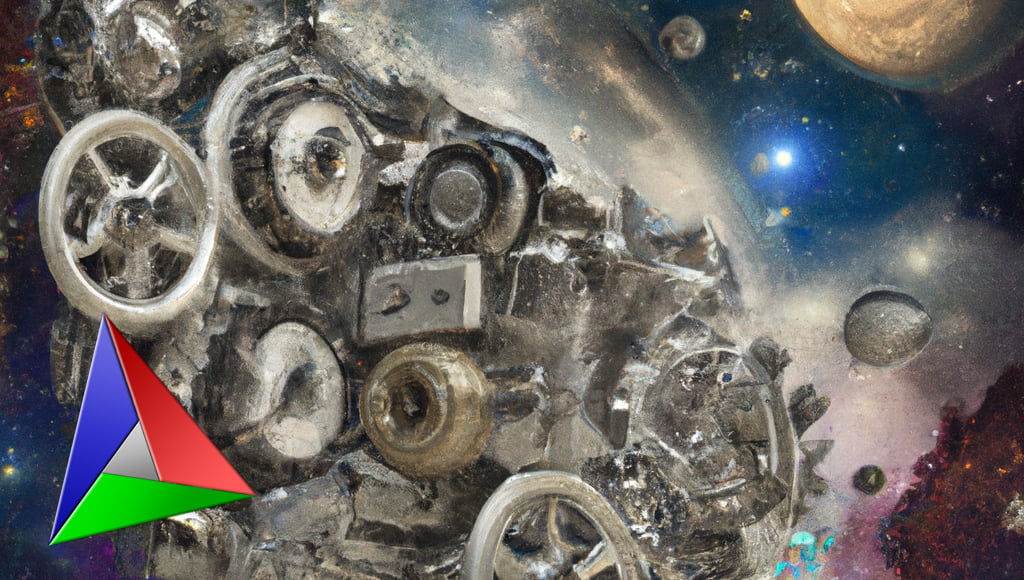
With CMake, this isn’t as difficult as many think. In fact, you only need to set three main variables: CMAKE_CXX_STANDARD
, CMAKE_CXX_STANDARD_REQUIRED
, and CMAKE_CXX_EXTENSIONS
.
Let’s Talk About The C++ Standard CMake Variables
Firstly, let’s discuss how CMake sets the standard throughout your project.
Essentially, every executable or library you declared in your CMakeSettings.txt
is a target. For example, creating executables in CMake will in turn generate a named target for it in CMake. Each target has properties set by CMake, and some of those properties relate to the C++ standard for the compilation:
CXX_STANDARD
dictates which standard version the target will be compiled with. Specifically, at the time of writing the possible values are{98, 11, 14, 17, 20, 23, 26}
. If you’re reading this from the far future, this list may have expanded.CXX_STANDARD_REQUIRED
is a boolean that makes the standard defined inCXX_STANDARD
a requirement. Therefore, configuring the project will not succeed if the compiler used does not support the standard you specified if this flag is set toOn
.CXX_EXTENSIONS
is another boolean value. Briefly speaking, it tells CMake whether compiler extensions (such as inline assembly) are allowed or not. For more portable code, set this value toOff
to ensure your code will compile in all compilers.
Setting these properties to targets can be done with the following snippets.
set_property(TARGET target PROPERTY CXX_STANDARD 17)
set_property(TARGET target PROPERTY CXX_STANDARD_REQUIRED On)
set_property(TARGET target PROPERTY CXX_EXTENSIONS Off)
As you can see, we set the standard of target
to 17, requiring that standard, and also turning extensions off.
The Recommended Approach To Set The C++ Standard In CMake
Although you can set the standard property on a “target” basis, essentially being able to have different standards for different parts of your codebase, it’s generally recommended not to.
Unless you have a very good reason, you probably want to set the same standard for every target in your project at once. Therefore, the CMAKE_*
global variables are very handy here.
Long story short, the CMake documentation specifies that the properties CXX_STANDARD
, CXX_STANDARD_REQUIRED
, and CXX_EXTENSIONS
have initial values. Specifically, they get initialised to the value of CMAKE_CXX_STANDARD
, CMAKE_CXX_STANDARD_REQUIRED
, and CMAKE_CXX_EXTENSIONS
respectively.
In other words, if you don’t specify these properties on a target, the default values of these properties will be set as the global variables mentioned above. Therefore, you can fully specify the standard settings like the example below.
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED On)
set(CMAKE_CXX_EXTENSIONS Off)
For this reason, stick to the code above when you can! In addition, make sure you call the CMake functions above straight after the project(...)
call, or before you create any targets.
When Should You Have Different Versions For Different Targets?
Unfortunately, there aren’t many reasons for having different standard versions in different parts of your codebase.
The C++ standard has always been backwards compatible with previous standard versions. Therefore, if your code compiles in C++14, rest assured it will also compile in C++20. So you can always set the standard as the latest standard your compiler supports.
However, you may have different compilers being used in your project. Therefore, they may not support the same C++ standards. In this case, it’s plausible that you may want to have different versions for different targets.
What Happens If You Don’t Set The C++ Standard In CMake?
In simple terms, the compiler will dictate what standard to use by default. You can check out this compiler standard defaults gist to find out what version is used by default in your compiler.
For example, for gcc 10.2
, the default version is 201402L
, and the first four digits indicate that it uses C++ 2014 standard (or 14
for CMAKE_CXX_STANDARD
).
Learning The CMake C++ Standards Settings By Video Example
If you’re still interested in seeing more action regarding setting the C++ compiler settings, the video below shows the usage, examples, and tips on using the CMake features for setting the C++ standard.
If you haven’t already done so, check out my Youtube channel, and if possible, subscribe as that helps out massively!
Further Reading & Notes
CXX_STANDARD
documentation on CMake’s website.CXX_STANDARD
documentation on CMake’s website.CXX_EXTENSIONS
documentation on CMake’s website.
All the properties and settings mentioned in this post show work for both executables and libraries created with CMake. Check out how to create executables using CMake, and while you’re at it, also learn how to create libraries with CMake.
Thanks for reading this post!
More importantly: do you have any questions, feedback, or suggestions? Spotted a typo? Feel free to post a comment below!
Be First to Comment