As my first post, I thought it would be interesting to dissect and analyse the simplest C++ program a person can write and compile. This post aims to teach beginners how the famous main
function works, why it is required by the compilers and what is represented by its return value.
The “main” Function In C++
If you have ever taken a beginners CPP course, you have undoubtedly come across the main
function. Unfortunately, very few of these so-called “beginners” courses do not go through the trouble of explaining what this function is and why it is necessary for a simple “Hello World!” program. In my opinion, skipping the details of this basic function causes beginner C++ developers to wonder what the weird syntax does, and more importantly, it misses out on the opportunity to explain an important part of an executable computer program: an entry point.
Stepping away from complaining about beginner courses, let’s have a look at the simplest main
function in C++ in the code block below, which is also the simplest compilable C++ program:
int main()
{
}
Note that, this is not a “Hello World!” tutorial, so the program above does not actually do much. However, the above code does show fundamental aspects of C++ and programming in general, namely function definitions, data types, and scope.
What Purpose Does “main” Serve?
If you have used a computer in the past, you have definitely come across an executable program. The very browser in which you are reading this post is itself an executable, whether you are using a mobile phone or a PC browser.
Once you click on your browser, the computer needs to know what part of the code to begin executing. This is where main
comes in, it is the entry point of the program and it must be defined if you are developing an executable. In fact, your executable will not even compile if the main
function is not defined!
However, if you are not writing an executable then you do not need to define the entry point. For example, if you are writing a CPP library to be included/linked in different projects, you don’t need to define a function named main
.
Diving Into The “main” Function Definition
In the snippet above, it is clear that main
is a pretty standard function that returns an integer (except there is no return
statement — which we will explain).
The Scope of main
And What It Means
As you probably know, main
is the name of the function and everything between the curly braces {}
is the code that will run when your program starts. The scope of main
is defined by these curly braces, meaning that everything in it belongs to main
and any variables/declarations you create can be referenced within this function.
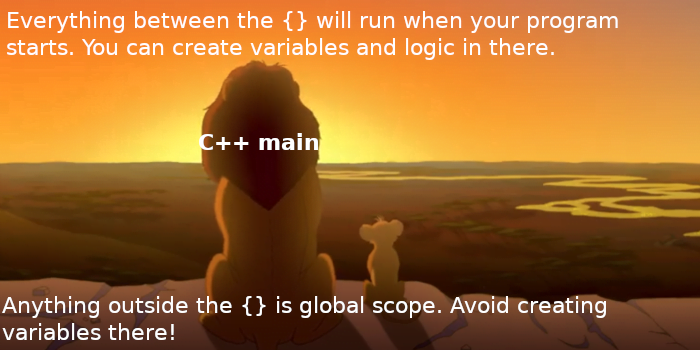
Return Type Of main
The return type of main must be int
. It indicates the error code your program will return when finished. Historically, the value 0
means no error, nothing unexpected happened during the execution of your code. Any other value (for example -1
or 29402348
) returned from main indicates that something wrong happened. A famous example is trying to access a value outside the bounds of a CPP vector, where your program will exit and return a value that is not 0
! We have all been there!
Aside from 0
meaning “OK” and non-zero values implying an error, there is no standard that maps the integer values to specific errors; error codes and meanings are left to be defined by you!
But why does the code snippet above does not have a return
statement? Well, in the exceptional case of the main
function, the compilers will automatically add a return 0;
to the end of it. Meaning that you could, if you wanted, add that line yourself. Or perhaps even return a non-zero value somewhere along your lines of code if something went wrong. Just to be clear, the previous code snippet can be also written in the following form:
int main()
{
return 0;
}
The above snippet does exactly the same as the previous one: simply nothing and returns no errors! A pretty simple program indeed.
To summarise, both snippets that we have seen so far define a function called main
which returns an int
, the error code. Everything inside the curly brackets ({}
) is the code that will run when you run your executable.
Slightly More Complex Definition Of The C++ main
Function
So far, we have seen the simplest version of main
, but it is not the only one.
The other important definition of main
is useful when you need to pass command-line arguments, or inputs, to your program. If you have used the command-line before (such as bash on Linux or cmd on Windows), you have probably seen examples of this technique used in popular tools, such as git.
An example of this is git push -f
. Here, git
is the executable you are calling, and push -f
are the command-line arguments you’re giving to git
.
In C++, the command-line arguments can be accessed with the following definition of main
:
int main(int argc, char** argv)
{
// Some code that makes use of the
// command-line arguments for example...
std::cout << "number of arguments given "
<< std::to_string(argc) << "\n";
std::cout << "First argument is "
<< argv[0];
}
The example above, although a little more complex than the simpler version of main
, is still basic. It shows the number of command-line arguments given to your program when called (the integer argc
), and displays the first argument through accessing the first element of the array argv
.
It is worth mentioning that the value of argc
will always be at least 1
. This is because the very first argument which is always indirectly passed to your executable is the name of the executable itself, or more specifically, the path to it. Hence, if the program you created is called “example.exe”, and you call it within the same directory with example.exe one two
, then the value of argc
will be 3
and the contents of argv
will be {"example.exe", "one", "two"}
.
Alternative Ways To Define main
In C++
You can write function definitions in different ways since modern CPP (C++ 11 and so on) came along. The snipped below shows an alternative definition of main
which achieves the same thing as the simpler version we previously saw.
auto main() -> int
{
// Your code goes here
}
For the command-line arguments case.
auto main(int argc, char** argv) -> int
{
// Your code goes here
}
The above definitions use the auto
keyword and trailing return
types, available in C++11 and later versions. Some people argue that this form of writing function definitions is more readable than the traditional “return type, name and scope” way. It focuses on the function name and makes all the function definitions easier to scan through!
TL;DR
The main
function is the entry point of your program; everything you add to it will execute when you call your program.
The return
type must be an integer by design, and anything else will not compile! You can define your own error codes, the only standard is that 0
means “no error”, and non-zero values mean “something wrong”.
Different people have different tastes. There are a couple of ways you can define main
. Whatever you pick, make sure you are consistent so your codebase does not end up looking like Frankenstein!
Did I miss anything? Any mistakes in my post? Feel free to post a comment with feedback!
[…] addition to improving a simple C++ program with the points made above, there are other motivations for using std::vector such […]
[…] simplest CPP program taken from a previous post and shown in the code box below is indeed not very useful. It will […]